Introduction
As technology advances, protecting our homes has become more important than ever. Systems for locking doors with fingerprint sensors provide an innovative way to improve the security of your house or place of business. Using easily accessible parts and Arduino technology, this blog article will walk you through the process of building a personalized Fingerprint Door Lock System. The “Fingerprint Door Lock Security System” by MArobotics uses Arduino Uno to provide a sophisticated security solution. This ingenious idea combines the accuracy of fingerprint recognition technology with the flexibility of Arduino to produce a strong and secure door entry system. MArobotics’ Fingerprint Door Lock improves security measures for homes and facilities by providing a complex yet approachable solution to modern door access management.
Components Required
- Drawing Board
- 3mm Acrylic Sheet
- Solderless Breadboard
- Arduino UNO
- I2C LCD Module
- 16×2 LCD Display
- Fingerprint Sensor
- Push Button x 4
- L298 Motor Driver
- Buzzer
- DVD Room Gate (assuming you mean a DVD drive or optical drive)
- Male to Male Jumper Wires
- Male to Female Jumper Wires
- Female DC Power Jack
- 5V 2Amp Power Adapter
Circuit Diagram
A 5V, 2A power adaptor regulates the circuit’s initial 220V power source, which supplies a steady DC supply for the whole apparatus. The central processing unit, or Arduino Uno, is in charge of coordinating the operations of the many parts. The 16×2 LCD is a crucial component of the circuit and is effectively controlled by an I2C module. The I2C module maximizes the use of the Arduino’s GPIO pins and streamlines the wiring. An essential part of biometric authentication, the fingerprint sensor is included into the system. It has a dedicated pin connection to the Arduino, which guarantees correct communication for fingerprint identification. Four strategically positioned push buttons, for features like deletion, system control, and enrollment, improve the user experience. The user’s engagement with the security system relies heavily on these buttons. The L298 motor driver, which has an H-bridge arrangement, is essential to the door’s actual functionality. The DVD Room Gate is operated by a single H-bridge, which replicates the opening and closing of the door. The motor driver is attached to the Arduino and is configured to operate continuously with its enable pin jumpered. It receives signals through In1 and In2. An Arduino pin 13 buzzer is attached to offer an auditory alarm system. Certain situations, including an unsuccessful attempt at fingerprint recognition, cause this buzzer to sound. The circuit also takes into consideration the power needs of the driver and the engine. The driver is supplied by the 5V output from the power adapter, while the motor receives a 5V supply directly. Carefully establishing the ground connections guarantees that every component has a common reference.
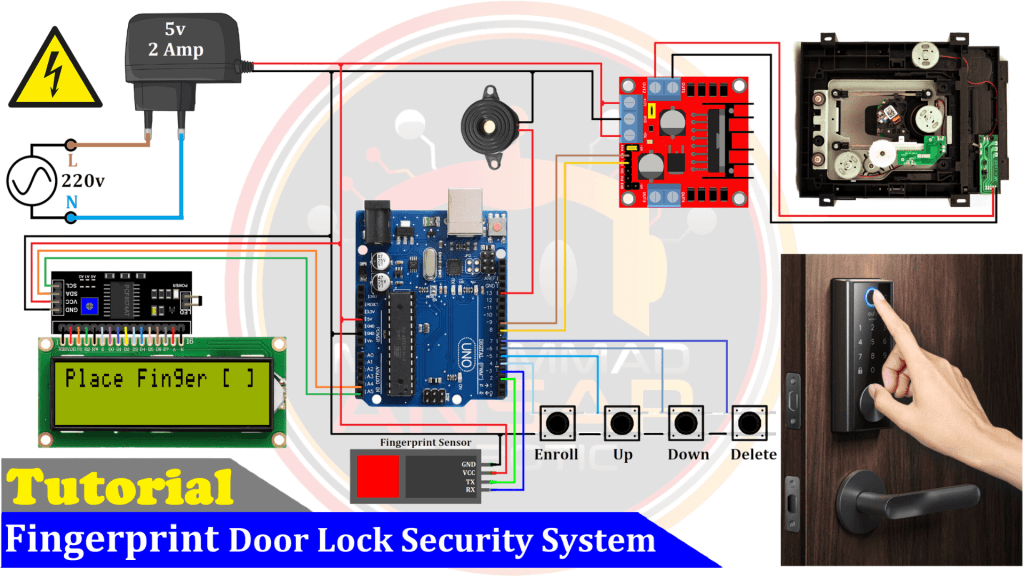
Arduino IDE Code
#include "LiquidCrystal_I2C.h"
LiquidCrystal_I2C lcd(0x27, 16, 2); // I2C address 0x27, 16 column and 2 rows
#include <SoftwareSerial.h>
SoftwareSerial fingerPrint(2, 3);
#include "Adafruit_Fingerprint.h"
uint8_t id;
Adafruit_Fingerprint finger = Adafruit_Fingerprint(&fingerPrint);
int total_id = 25; // set value for total fingerprint sensor Storage Capacity
int set_time=8; //Set the timer here for how long the door should stay open
#define bt_enroll 4
#define bt_up 5
#define bt_down 6
#define bt_delete 7
#define door_C_pin 8
#define door_O_pin 9
#define buzzer 13 // choose the pin for the buzzer
void setup(){ // put your setup code here, to run once
Serial.begin(9600);
finger.begin(57600);
pinMode(bt_enroll, INPUT_PULLUP);
pinMode(bt_up, INPUT_PULLUP);
pinMode(bt_down, INPUT_PULLUP);
pinMode(bt_delete, INPUT_PULLUP);
pinMode(door_O_pin,OUTPUT);
pinMode(door_C_pin,OUTPUT);
pinMode(buzzer,OUTPUT); // declare buzzer as output
lcd.init();// initialize the lcd
lcd.backlight();
lcd.setCursor(0,0);
lcd.print("Security System");
lcd.setCursor(0,1);
lcd.print("by Finger Print");
delay(2000); // Waiting for a while
lcd.clear();
lcd.print("Finding Module");
lcd.setCursor(0,1);
delay(1000);
if(finger.verifyPassword()){Serial.println("Found fingerprint sensor!");
lcd.clear();
lcd.print("Found Module ");
}
else{Serial.println("Did not find fingerprint sensor :(");
lcd.clear();
lcd.print("module not Found");
lcd.setCursor(0,1);
lcd.print("Check Connections");
while (1);
}
digitalWrite(door_O_pin, LOW); // Door Open
digitalWrite(door_C_pin, HIGH);// Door Close
delay(2000); // Waiting for a while
digitalWrite(door_O_pin, LOW); // Door Open
digitalWrite(door_C_pin, LOW); // Door Close
lcd.clear();
}
void loop(){
lcd.setCursor(0,0);
lcd.print("Place Finger [ ]");
lcd.setCursor(0,1);
lcd.print(" ");
int result=getFingerprintIDez();
if(result>0){
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
lcd.clear();
lcd.print("Please Wait... ");
lcd.setCursor(0,1);
lcd.print(" Door is Opening");
digitalWrite(door_O_pin, HIGH);// Door Open
digitalWrite(door_C_pin, LOW); // Door Close
delay(2000); // Waiting for a while
digitalWrite(door_O_pin, LOW); // Door Open
digitalWrite(door_C_pin, LOW); // Door Close
lcd.clear();
lcd.print(" Welcome ");
lcd.setCursor(0,1);
lcd.print(" Door is Open ");
for(int i=0;i<set_time;i++){delay(1000);}
lcd.clear();
lcd.print("Please Wait... ");
lcd.setCursor(0,1);
lcd.print(" Door is Closing");
digitalWrite(door_O_pin, LOW); // Door Open
digitalWrite(door_C_pin, HIGH);// Door Close
delay(2000); // Waiting for a while
digitalWrite(door_O_pin, LOW); // Door Open
digitalWrite(door_C_pin, LOW); // Door Close
delay(100);
lcd.clear();
return;
}
checkKeys();
delay(500);
}
void checkKeys(){
if(digitalRead(bt_enroll) == 0){
lcd.clear();
lcd.print("Please Wait");
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
delay(1000);
while(digitalRead(bt_enroll) == 0);
Enroll();
}
else if(digitalRead(bt_delete) == 0){
lcd.clear();
lcd.print("Please Wait");
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
delay(1000);
delet();
}
}
void Enroll(){
int count=0;
lcd.clear();
lcd.print("Enroll Finger ");
lcd.setCursor(0,1);
lcd.print("Location:");
while(1){
lcd.setCursor(9,1);
lcd.print(count);
lcd.print(" ");
if(digitalRead(bt_up) == 0){
count++;
if(count>25)count=0;
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
}
else if(digitalRead(bt_down) == 0){
count--;
if(count<0)count=25;
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
}
else if(digitalRead(bt_enroll) == 0){
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
id=count;
getFingerprintEnroll();
return;
}
else if(digitalRead(bt_delete) == 0){
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
return;
}
}
}
void delet(){
int count=0;
lcd.clear();
lcd.print("Delete Finger ");
lcd.setCursor(0,1);
lcd.print("Location:");
while(1){
lcd.setCursor(9,1);
lcd.print(count);
lcd.print(" ");
if(digitalRead(bt_up) == 0){
count++;
if(count>25)count=0;
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
}
else if(digitalRead(bt_down) == 0){
count--;
if(count<0)count=25;
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
}
else if(digitalRead(bt_delete) == 0){
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
id=count;
deleteFingerprint(id);
return;
}
else if(digitalRead(bt_enroll) == 0){
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
return;
}
}
}
uint8_t getFingerprintEnroll(){
int p = -1;
lcd.clear();
lcd.print("finger ID:");
lcd.print(id);
lcd.setCursor(0,1);
lcd.print("Place Finger");
delay(2000);
while (p != FINGERPRINT_OK)
{
p = finger.getImage();
switch (p)
{
case FINGERPRINT_OK:
Serial.println("Image taken");
lcd.clear();
lcd.print("Image taken");
break;
case FINGERPRINT_NOFINGER:
Serial.println("No Finger");
lcd.clear();
lcd.print("No Finger");
break;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
lcd.clear();
lcd.print("Comm Error");
break;
case FINGERPRINT_IMAGEFAIL:
Serial.println("Imaging error");
lcd.clear();
lcd.print("Imaging Error");
break;
default:
Serial.println("Unknown error");
lcd.clear();
lcd.print("Unknown Error");
break;
}
}
// OK success!
p = finger.image2Tz(1);
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image converted");
lcd.clear();
lcd.print("Image converted");
break;
case FINGERPRINT_IMAGEMESS:
Serial.println("Image too messy");
lcd.clear();
lcd.print("Image too messy");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
lcd.clear();
lcd.print("Comm Error");
return p;
case FINGERPRINT_FEATUREFAIL:
Serial.println("Could not find fingerprint features");
lcd.clear();
lcd.print("Feature Not Found");
return p;
case FINGERPRINT_INVALIDIMAGE:
Serial.println("Could not find fingerprint features");
lcd.clear();
lcd.print("Feature Not Found");
return p;
default:
Serial.println("Unknown error");
lcd.clear();
lcd.print("Unknown Error");
return p;
}
Serial.println("Remove finger");
lcd.clear();
lcd.print("Remove Finger");
delay(2000);
p = 0;
while (p != FINGERPRINT_NOFINGER) {
p = finger.getImage();
}
Serial.print("ID "); Serial.println(id);
p = -1;
Serial.println("Place same finger again");
lcd.clear();
lcd.print("Place Finger");
lcd.setCursor(0,1);
lcd.print(" Again");
while (p != FINGERPRINT_OK) {
p = finger.getImage();
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image taken");
break;
case FINGERPRINT_NOFINGER:
Serial.print(".");
break;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
break;
case FINGERPRINT_IMAGEFAIL:
Serial.println("Imaging error");
break;
default:
Serial.println("Unknown error");
return;
}
}
// OK success!
p = finger.image2Tz(2);
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image converted");
break;
case FINGERPRINT_IMAGEMESS:
Serial.println("Image too messy");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
return p;
case FINGERPRINT_FEATUREFAIL:
Serial.println("Could not find fingerprint features");
return p;
case FINGERPRINT_INVALIDIMAGE:
Serial.println("Could not find fingerprint features");
return p;
default:
Serial.println("Unknown error");
return p;
}
// OK converted!
Serial.print("Creating model for #"); Serial.println(id);
p = finger.createModel();
if (p == FINGERPRINT_OK) {
Serial.println("Prints matched!");
} else if (p == FINGERPRINT_PACKETRECIEVEERR) {
Serial.println("Communication error");
return p;
} else if (p == FINGERPRINT_ENROLLMISMATCH) {
Serial.println("Fingerprints did not match");
return p;
} else {
Serial.println("Unknown error");
return p;
}
Serial.print("ID "); Serial.println(id);
p = finger.storeModel(id);
if (p == FINGERPRINT_OK) {
Serial.println("Stored!");
lcd.clear();
lcd.print("Stored!");
delay(2000);
} else if (p == FINGERPRINT_PACKETRECIEVEERR) {
Serial.println("Communication error");
return p;
} else if (p == FINGERPRINT_BADLOCATION) {
Serial.println("Could not store in that location");
return p;
} else if (p == FINGERPRINT_FLASHERR) {
Serial.println("Error writing to flash");
return p;
}
else{
Serial.println("Unknown error");
return p;
}
}
int getFingerprintIDez(){
uint8_t p = finger.getImage();
if (p != FINGERPRINT_OK)
return -1;
p = finger.image2Tz();
if (p != FINGERPRINT_OK)
return -1;
p = finger.fingerFastSearch();
if (p != FINGERPRINT_OK){
lcd.clear();
lcd.print("Finger Not Found");
lcd.setCursor(0,1);
lcd.print("Try Later ");
for(int x=0; x<10; x++){
digitalWrite(buzzer, HIGH);
delay(300);
digitalWrite(buzzer, LOW);
delay(100);
}
return -1;
}
// found a match!
Serial.print("Found ID #");
Serial.print(finger.fingerID);
return finger.fingerID;
}
uint8_t deleteFingerprint(uint8_t id){
uint8_t p = -1;
lcd.clear();
lcd.print("Please wait");
p = finger.deleteModel(id);
if(p == FINGERPRINT_OK) {Serial.println("Deleted!");
lcd.clear();
lcd.print("Figer Deleted");
lcd.setCursor(0,1);
lcd.print("Successfully");
delay(1000);
}else{Serial.print("Something Wrong");
lcd.clear();
lcd.print("Something Wrong");
lcd.setCursor(0,1);
lcd.print("Try Again Later");
delay(2000);
lcd.clear();
return p;
}
}
Explanation
The LiquidCrystal_I2C library header file and two Adafruit fingerprint library header files are included at the start of the code. These are essential for operating the I2C-connected LCD and interacting with the fingerprint sensor. The startup, setup, and looping tasks common to Arduino programs are handled by the code’s structure. First, it initializes the LiquidCrystal_I2C library for the attached LCD and initializes the Adafruit fingerprint sensor library. The code needs to know the module’s I2C address since the LCD uses the I2C protocol to function. The code must accurately provide this address. It is advised to see a different video on how to use an I2C module in order to retrieve and configure this address. total_id: A variable that indicates the fingerprint sensor’s total storage capacity. The datasheet for the sensor contains standards that should be followed when adjusting this value. set_time: Indicates how long a door stays open following a successful fingerprint recognition. Depending on the intended open time, change this value. Make sure the Arduino IDE settings are set up correctly before uploading the code to the Arduino Uno. Choose the proper COM port and board (Arduino Uno). After setting up, send the code to the Arduino. Go to “Board” > “Tools” > “Arduino Uno.” Choose the relevant COM port by going to “Tools” > “Port.” Click the “Upload” button to upload the code. The Arduino IDE’s “Done uploading” notification verifies that the upload procedure has finished. This shows that the code has been successfully sent to the Arduino Uno, indicating that testing of the system is now possible.
Hardware Testing
The Fingerprint Door Lock System exhibits flawless operation throughout hardware testing. When there are initially no fingerprints saved, the system warns about the lack of authentication. Clicking the enroll button, choosing an ID, and putting your finger there to confirm are the steps in the enrolling procedure. The door will then open for a certain amount of time when the saved fingerprint is used to gain entrance. Multiple fingerprints can be supported by the system, enabling users to enroll new IDs and remove old ones. Crucially, the illustration highlights how important it is to configure the code based on the capabilities of the fingerprint sensor. By allowing or refusing access based on fingerprints that have been saved, the system demonstrates its efficacy and highlights its suitability for safe and customized entrance control.
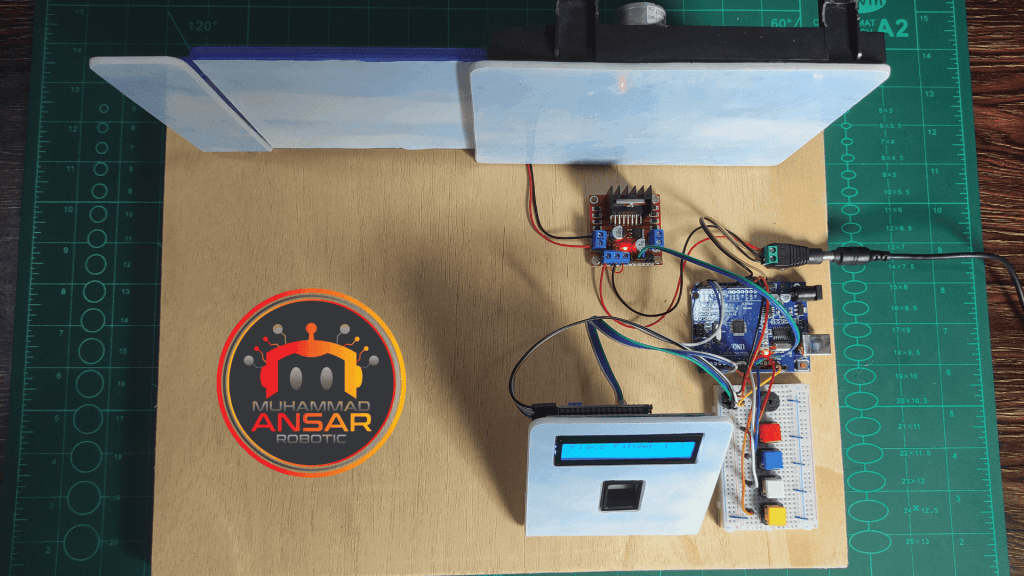
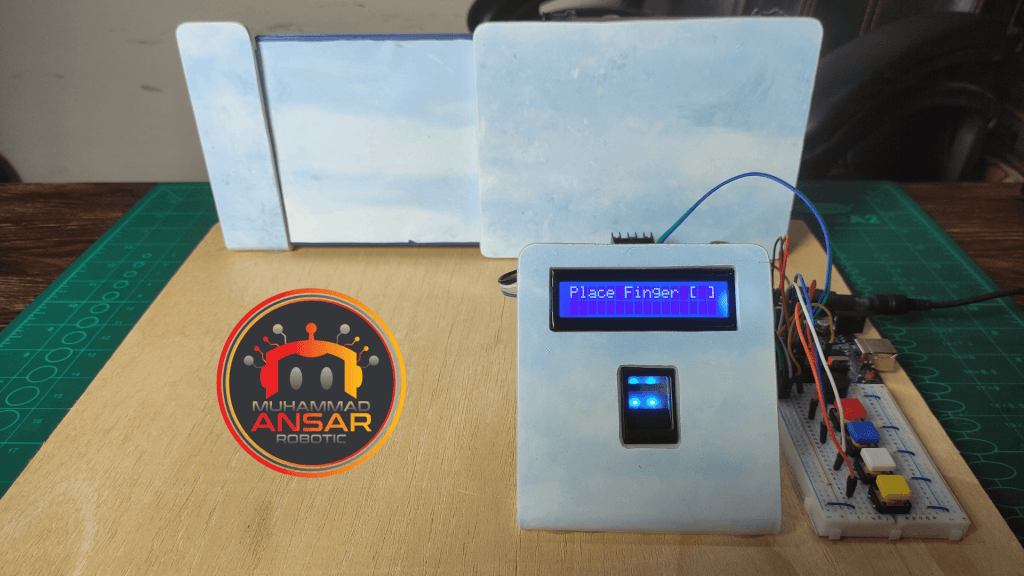
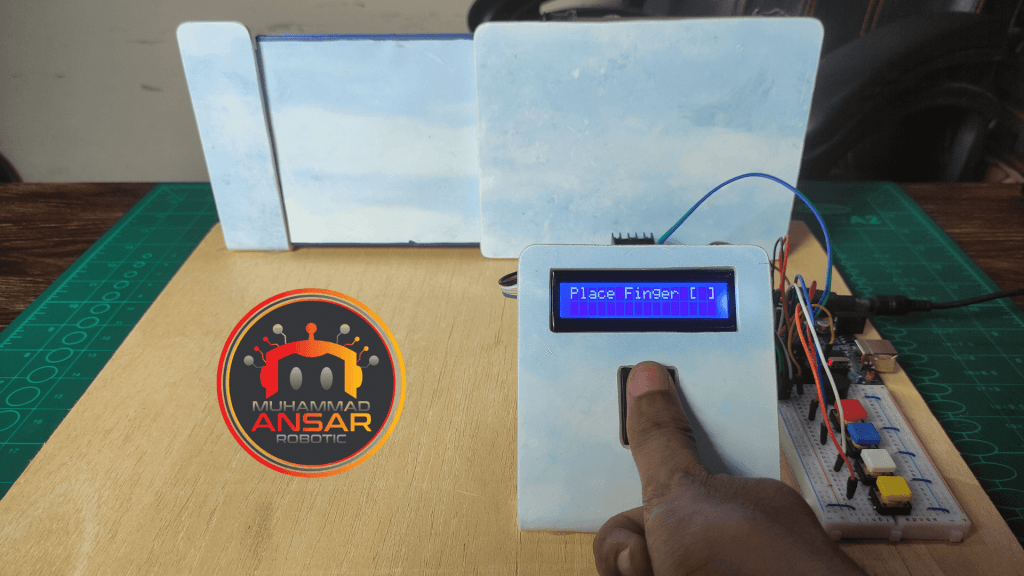
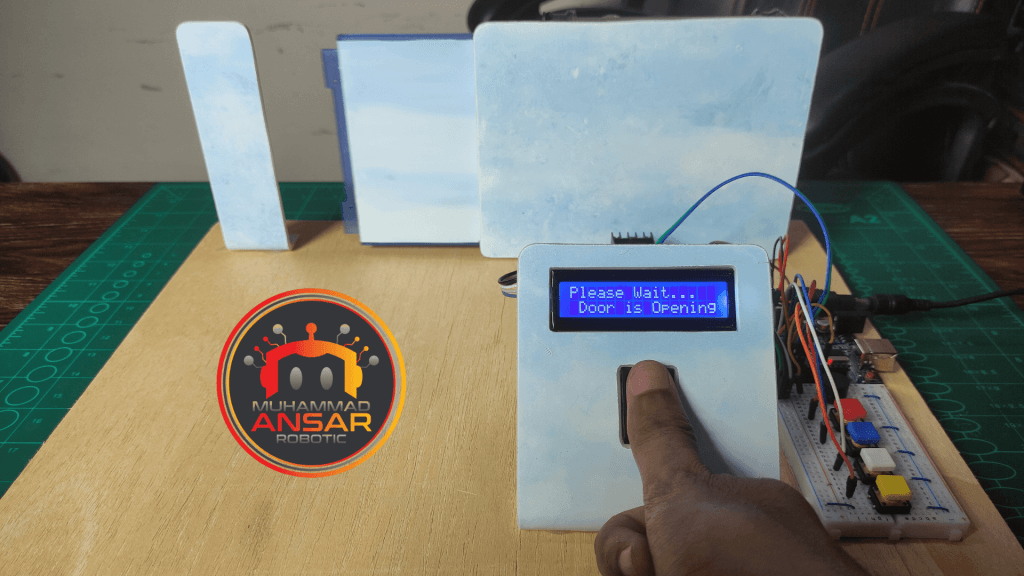
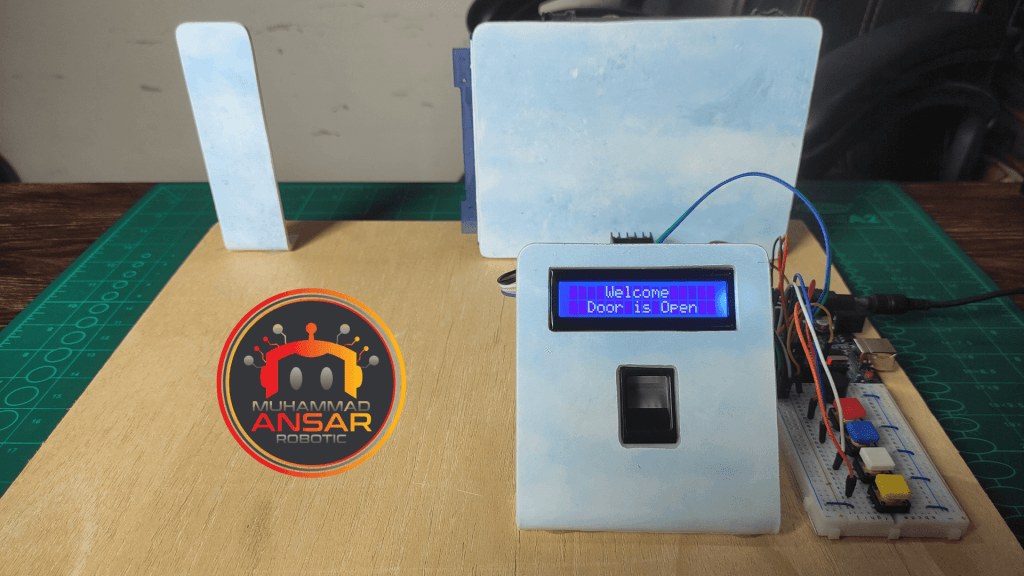
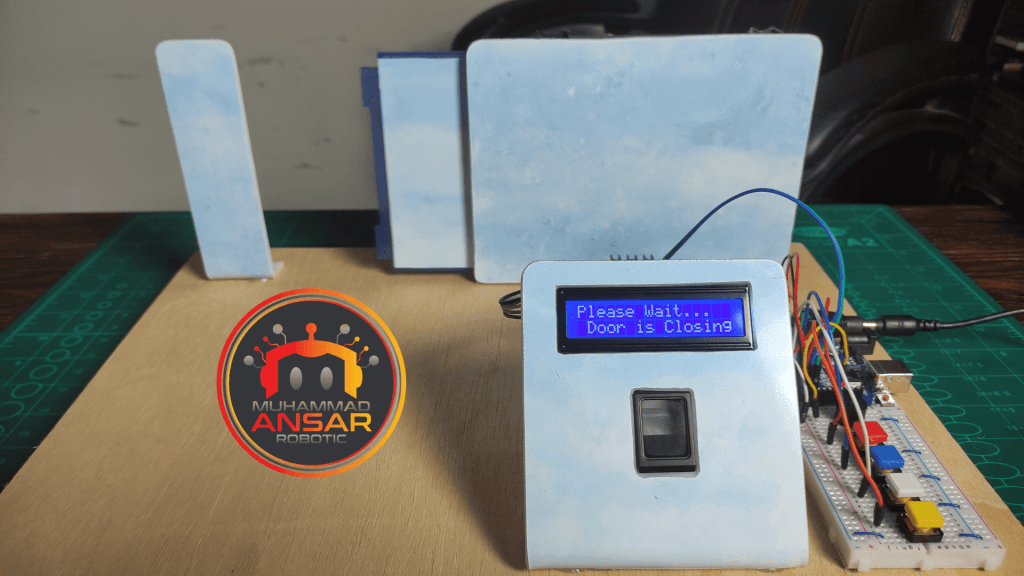
Conclusion
Best wishes! You’ve successfully used Arduino technology and common components to develop a Fingerprint Door Lock System. This project proves the capabilities of do-it-yourself electronics while also improving the security of your area. Enjoy the ease and peace of mind that come with a customized fingerprint sensor-based door lock by expanding and customizing this project to fit your unique needs.
Leave a Reply