Introduction
MArobotics created the Arduino and MQ-3 Sensor-based Alcohol Detector, a sophisticated system for detecting the presence of alcohol vapor in the environment around it. The project makes use of a MQ-3 gas sensor, which is particularly sensitive to alcohol vapors, and an Arduino microcontroller for processing the data. The combination of these components allows for the development of an efficient and portable alcohol detection system, resulting in a useful tool for applications such as breathalyzer devices and alcohol content monitoring in a variety of scenarios. MArobotics’ initiative offers a practical and easily available option for anyone seeking to deploy alcohol detection technology in a variety of settings. In order to ensure public safety, alcohol detectors are essential, particularly when it comes to averting drunk driving-related tragedies. We’ll walk you through the steps of building an alcohol detector with an Arduino and a MQ-3 sensor in this blog article. This project can assist you in comprehending the fundamentals of alcohol detection and be a useful resource for keeping an eye on alcohol levels in a variety of settings.
Components Required
- Solderless Breadboard
- Arduino UNO
- 16×2 LCD Display
- MQ-3 Sensor
- 4.7k Resistor
- 10k Variable Resistor
- 100R Resistor x 3
- Green LED
- Red LED
- Buzzer
- Male to Male Jumper Wires
- Hard Jumper Wire
- Battery Clip
- 9V Battery
Proteus Simulation
Proteus 8.12, a virtual environment, is used for the simulation in order to verify and test our configuration before putting it into hardware. An Arduino Uno, a MQ-3 alcohol detection sensor, a 16×2 LCD display, a 10k variable resistor to modify LCD contrast, a buzzer to sound alarms, and two LEDs (red and green) to show the alcohol content status are the parts used in the simulation. In addition, the circuit design makes use of two 100-ohm resistors to regulate the LEDs. Open the simulation file on Proteus 8. The microcontroller used is Arduino UNO. MQ-3 gas sensor is used. Vcc is connected to the MQ-3 Vcc terminal. Its ground pin (GND) is connected to ground. The output of the MQ-3 sensor is connected to the A0 pin. Positive terminal of a buzzer is connected to pin 13 of the Arduino UNO. Its negative terminal is connected to ground with a 10k resistor in series. A red LED is connected to pin 9 of the Arduino UNO. A green LED is connected to pin 8 of the Arduino UNO. A 16×2 LCD is used as a display. On the first line, it displays BAC value in mg/litre. In second line, it displays drunk or normal.
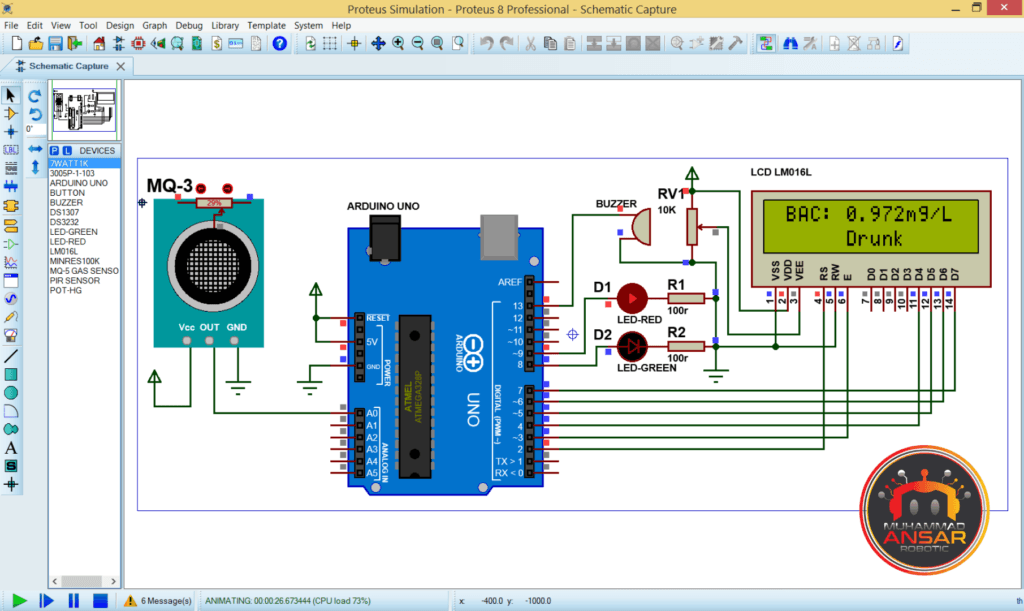
The address of the created hex file must be transferred in order to launch the simulation after the Arduino code has been built in the Arduino IDE. The Arduino component in the Proteus simulation is then updated with this address. The simulation shows the status (either “Normal” or “Drunk”) on the second line of the LCD and the amount of alcohol in milligrams per liter on the first. The green LED stays on and “Normal” is shown when the alcohol reading is less than 0.8. Nevertheless, “Drunk” appears along with the red LED and buzzer sound a warning when the number rises above 0.8. You can experiment with the simulation’s alcohol threshold value to see how the system reacts to various alcohol concentrations. The system goes back to “Normal” mode and the green LED illuminates while the red LED and alert are turned off whenever the alcohol level is within normal limits. Prior to implementing the alcohol detector on hardware, it is imperative to validate its operation in this simulated environment.
Circuit Diagram
A 9-volt battery is used as the energy source to run the complete system. An Arduino Uno is the central component of the arrangement, utilized for both component control and monitoring. The MQ-3 sensor is included into the circuit to perform the vital function of alcohol detection. This sensor is essential to precisely measuring alcohol concentrations. The Arduino is linked to a 16×2 LCD display to give immediate feedback and real-time data. To ensure that the presented data is clearly visible, the LCD’s contrast level is adjusted using a 10-kilohm potentiometer. The lighting is controlled by two pins on the LCD, pins 15 and 16. Pin 15 has a 100-ohm resistor connected in series with the voltage from the positive source to control the backlight’s intensity, whereas Pin 16 is connected straight to ground. To further give visual alerts, two LEDs—one red and one green—are included into the circuit. These LEDs’ positive terminals are connected to the Arduino Uno, and their negative terminals are connected to ground via a series of 100-ohm resistors. By ensuring that the LEDs get a regulated current flow, these resistors shield the LEDs from overcurrent-related burnout. When the alcohol content rises beyond the predetermined level, a buzzer included into the design will sound a warning.
Arduino IDE Code
#include <LiquidCrystal.h> //Libraries
LiquidCrystal lcd(2, 3, 4, 5, 6, 7); //Arduino pins to lcd
#define sensor_pin A0
#define G_led 8
#define R_led 9
#define buzzer 13
float adcValue=0, val=0, mgL=0;
void setup(){// put your setup code here, to run once
pinMode(sensor_pin, INPUT);
pinMode(R_led,OUTPUT); // declare Red LED as output
pinMode(G_led,OUTPUT); // declare Green LED as output
pinMode(buzzer,OUTPUT); // declare Buzzer as output
lcd.begin(16, 2); // Configura lcd numero columnas y filas
lcd.clear();
lcd.setCursor (0,0);
lcd.print(" Welcome To ");
lcd.setCursor (0,1);
lcd.print("Alcohol Detector");
delay(2000);
lcd.clear();
}
void loop(){
adcValue=0;
for(int i=0;i<10;i++){
adcValue+= analogRead(sensor_pin);
delay(10);
}
val = (adcValue/10) * (5.0/1024.0);
mgL = 0.67 * val;
lcd.setCursor(0, 0);
lcd.print(" BAC: ");
lcd.print(mgL,3);
lcd.print("mg/L ");
lcd.setCursor(0, 1);
if(mgL>0.8){
lcd.print(" Drunk ");
digitalWrite(buzzer, HIGH);
digitalWrite(G_led, LOW); // Turn LED off.
digitalWrite(R_led, HIGH); // Turn LED on.
delay(300);
}else{
lcd.print(" Normal ");
digitalWrite(G_led, HIGH); // Turn LED on.
digitalWrite(R_led, LOW); // Turn LED off.
}
digitalWrite(buzzer, LOW);
delay(100);
}
Explanation
To communicate with the 16×2 LCD display, we first include the “LiquidCrystal.h” library in the Arduino code for our Alcohol Detector project. Pins 2 through 7 for the LCD, pin A0 for the MQ-3 alcohol sensor, and pins 8, and 9 for the green and red LEDs, respectively, are initialized next. The buzzer pin is marked at pin 13. After that, variables are declared to hold computed values and sensor data using float data types. The pins for the sensor, LEDs, and buzzer are specified as input or output in the setup function, which comes next. After initializing and clearing the LCD, a welcome message is shown for a 2000 ms delay.
A for loop is utilized in the code’s main loop to collect 20 analog samples from the MQ-3 sensor. To provide a precise measurement of the alcohol content, these samples are averaged. The number is then converted, taking into account the properties of the sensor, to milligrams per liter (mg/L). The LCD shows the alcohol content in milligrams per liter on the first line and indicates “Drunk” on the second line when the concentration is higher than 0.8 mg/L. The buzzer sounds, the green LED goes off, and the red LED turns on for a 300-millisecond alert all at once.
The LCD shows “Normal,” the red LED is off, and the green LED is on if the alcohol content is less than 0.8 mg/L. During this circumstance, there is a 100-millisecond delay and the buzzer stays off. The code loops back and forth for a hundred milliseconds, making sure that the alcohol content is continuously monitored. The buzzer sounds for 300 milliseconds when the concentration rises beyond the 0.8 mg/L threshold. Thereafter, there is a 100 millisecond wait before the loop repeats. When the code is prepared, it is uploaded to the Arduino Uno by choosing the proper board and port in the Arduino IDE.
Hardware Testing
It’s time to test the hardware when you’ve finished building the circuit and uploaded the code to your Arduino. Using the 9V battery to power up your setup, check the LCD display for readings on alcohol concentration. The system can also be tested by subjecting it to alcohol fumes. Verify that when alcohol consumption beyond the predetermined threshold, the buzzer and LEDs react accordingly.
Conclusion
An instructive and useful project is making an alcohol detector with an Arduino and a MQ-3 sensor. It may be used to many different things, such as public safety instruments and personal safety equipment. This project emphasizes the value of safely monitoring alcohol use in addition to showing how to link sensors and displays with Arduino. You may help improve community safety and get better knowledge of alcohol detection technologies by using this tutorial.
3 responses to “Arduino And MQ-3 Sensor Based Alcohol Detector”
How did you come up with the value 0.67 used in your mg/L calculation?
Very nice
thank you
Leave a Reply Cancel reply